Laravel 5 :(5)Migrations
Laravel 範例實作
此筆記主要紀錄學習以下 Laravel 的範例教學
Migrations
你可以用 Migrations 來建立或修改 database tables
使用資料庫
首先建立一個空的資料庫larashop
並修改/config/database.php
除了修改/config/database.php
外
記得跟目錄下的.env
也要一起改
1 | DB_CONNECTION=mysql |
Artisan migration 指令
當你使用Artisan migration 指令
時
會建立檔案在/database/migrations
底下
1.首先在跟目錄下執行1
php artisan migrate:install
成功會出現1
Migration table created successfully.
如果出現以下訊息,檢查/config/database.php
和.env
是否修改正確1
Access denied for user 'homestead'@'localhost' (using password: YES)
執行完此指令後會在larashop
資料庫下面建立一個資料表migrate
接著在執行
1 | php artisan make:migration create_drinks_table |
會在database/migrations/
底下多出一個檔案
1 | 2017_01_24_133914_create_drinks_table.php |
內容為
1 | class CreateDrinksTable extends Migration |
Migration 結構
將2017_01_24_133914_create_drinks_table.php
修改為
1 | class CreateDrinksTable extends Migration { |
再來執行
1 | php artisan migrate |
會出現
1 | Migrated: 2014_10_12_000000_create_users_table |
此時你的資料庫會出現
1 | larashop |
Laravel migration rollback
你可以執行以下指令來回復上一次動作
1 | php artisan migrate:rollback |
所以執行後資料庫會變為
1 | larashop |
再次執行以下指令建立資料表
1 | php artisan migrate |
Migration 操作
接下來要建立employees table
並插入亂數
亂數插入可參考 Faker Library,教學參考
首先執行
1 | php artisan make:migration employees |
修改database/migrations/2017_01_24_151737_employees.php
1 | class Employees extends Migration |
接著執行
1 | php artisan migrate |
此時你的資料庫會出現
1 | larashop |
點下employees
或執行SELECT * FROM employees;
可以看到裡面有我們插入的假帳號資訊
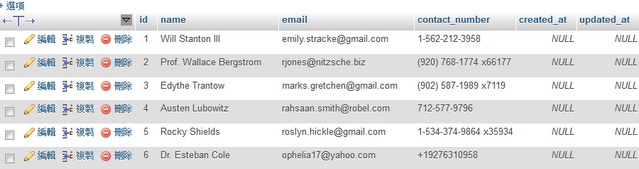
新增/刪除資料表欄位
資料表的欄位也會跟著變動
現在我們想要加一個gender
欄位到contact_number
之後
1 | php artisan make:migration add_gender_to_employees --table=employees |
此時會新增\database\migrations\2017_01_24_152951_add_gender_to_employees.php
-table=employees
表示此動作是在作用在employees
資料表
修改2017_01_24_152951_add_gender_to_employees.php
為
1 | class AddGenderToEmployees extends Migration |
接下來要安裝Database Abstract Layer DDBAL
,官網
在跟目錄打開composer.json
1 | "require": { |
修改完後執行composer update
composer 會開始下載檔案到Composer\files\doctrine
此為 composer 安裝目錄,不是在網站專案中
接著執行
1 | php artisan make:migration modify_gender_in_employees --table=employees |
此時會新增\database\migrations\2017_01_24_155118_modify_gender_in_employees.php
然後修改此檔案為
1 | class ModifyGenderInEmployees extends Migration |
最後執行
1 | php artisan migrate |
現在把gender
欄位改為可以為空的欄位
1 | php artisan make:migration make_gender_null_in_employees --table=employees |
修改2017_01_24_165034_make_gender_null_in_employees.php
1 | class MakeGenderNullInEmployees extends Migration |
執行php artisan migrate
你可以發現gender
欄位屬性改變
1 | 空值 預設值 |
使用 foreign key
我們想要將employees
中的人以departments
來分類
先建立depts
檔案
1 | php artisan make:migration depts |
修改2017_01_24_165501_depts.php
1 | class Depts extends Migration |
執行php artisan migrate
建立修改欄位檔案
1 | php artisan make:migration add_dept_id_in_employees --table=employees |
修改2017_01_24_170042_add_dept_id_in_employees.php
1 | class AddDeptIdInEmployees extends Migration |
執行php artisan migrate
輸入php artisan make:seeder DrinksTableSeeder
打開database\seeds\DrinksTableSeeder.php
修改為
1 | class DrinksTableSeeder extends Seeder { |
執行php artisan db:seed --class=DrinksTableSeeder
資料表drinks
會增加一個資料
1 | id name comments rating brew_date created_at updated_at |
實際練習
我們需要建立以下 4 個資料表
1 | 以下欄位所有資料表都要有 |
執行以下指令
1 | php artisan make:migration create_posts_table |
修改 4 個檔案
- 2017_01_24_172304_create_posts_table.php
1 | class CreatePostsTable extends Migration { |
2.2017_01_24_172307_create_products_table.php1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31class CreateProductsTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up() {
Schema::create('products', function (Blueprint $table) {
$table->increments('id');
$table->string('name', 255)->unique();
$table->string('title', 140);
$table->string('description', 500);
$table->integer('price');
$table->integer('category_id');
$table->integer('brand_id');
$table->timestamps();
$table->string('created_at_ip');
$table->string('updated_at_ip');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down() {
Schema::drop('products');
}
}
3.2017_01_24_172310_create_categories_table.php1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26class CreateCategoriesTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up() {
Schema::create('categories', function (Blueprint $table) {
$table->increments('id');
$table->string('name', 255)->unique();
$table->timestamps();
$table->string('created_at_ip');
$table->string('updated_at_ip');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down() {
Schema::drop('categories');
}
}
4.2017_01_24_172314_create_brands_table.php1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26class CreateBrandsTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up() {
Schema::create('brands', function (Blueprint $table) {
$table->increments('id');
$table->string('name', 255)->unique();
$table->timestamps();
$table->string('created_at_ip');
$table->string('updated_at_ip');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down() {
Schema::drop('brands');
}
}
執行php artisan migrate
加入假資料
1 | php artisan make:seeder ProductsTableSeeder |
修改檔案
1.ProductsTableSeeder.php1
2
3
4
5
6
7
8
9
10
11
12
13
14class ProductsTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('products')->insert(['name' => 'Mini skirt black edition', 'title' => 'Mini skirt black edition','description' => 'Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna','price' => 35,'category_id' => 1,'brand_id' => 1,]);
DB::table('products')->insert(['name' => 'T-shirt blue edition', 'title' => 'T-shirt blue edition','description' => 'Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna','price' => 64,'category_id' => 2,'brand_id' => 3,]);
DB::table('products')->insert(['name' => 'Sleeveless Colorblock Scuba', 'title' => 'Sleeveless Colorblock Scuba','description' => 'Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna','price' => 13,'category_id' => 3,'brand_id' => 2,]);
}
}
2.CategoriesTableSeeder.php1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16class CategoriesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('categories')->insert(['name' => 'MENS']);
DB::table('categories')->insert(['name' => 'WOMENS']);
DB::table('categories')->insert(['name' => 'KIDS']);
DB::table('categories')->insert(['name' => 'FASHION']);
DB::table('categories')->insert(['name' => 'CLOTHING']);
}
}
3.BrandsTableSeeder.php1
2
3
4
5
6
7
8
9
10
11
12
13
14class BrandsTableSeeder extends Seeder {
/**
* Run the database seeds.
*
* @return void
*/
public function run() {
DB::table('brands')->insert(['name' => 'ACNE']);
DB::table('brands')->insert(['name' => 'RONHILL']);
DB::table('brands')->insert(['name' => 'ALBIRO']);
DB::table('brands')->insert(['name' => 'ODDMOLLY']);
}
}
重點
1 | // 建立資料表 migrate |